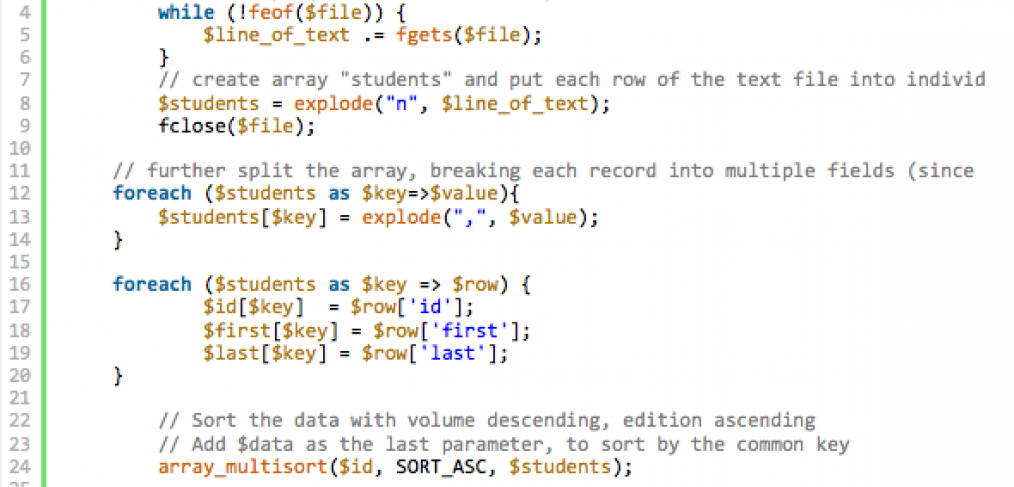
Simple PHP program to read a file and output the contents.
I’m taking a database class at California State University, Fullerton. This class involves learning PHP and MySQL and I already had my first assignment posted. The assignment is to read a text file containing student names with scrambled IDs, output the contents of the file in chunks of 5 records each. This is simple but it is just a start of the semester.
You can view the finished program here
The main code of the PHP program follows (description of what the code does is marked by “//”) :
[sourcecode language=”php”]
<?php
// open and read the file
$file = fopen("classlist.txt", "r");
while (!feof($file)) {
$line_of_text .= fgets($file);
}
// create array "students" and put each row of the text file into individual student record
$students = explode("n", $line_of_text);
fclose($file);
// further split the array, breaking each record into multiple fields (since there is ID, first and last name)
foreach ($students as $key=>$value){
$students[$key] = explode(",", $value);
}
foreach ($students as $key => $row) {
$id[$key] = $row[‘id’];
$first[$key] = $row[‘first’];
$last[$key] = $row[‘last’];
}
// Sort the data with volume descending, edition ascending
// Add $data as the last parameter, to sort by the common key
array_multisort($id, SORT_ASC, $students);
//define a function that will print a table row with the record fields
function print_row($value) {
print("<tr><td>$value[0]</td><td>$value[2]</td><td>$value[1]</td></tr>n");
}
// print a table header
echo("<table border=1><col width="50" /><col width="200" /><col width="150" /><tr><th>CWID</th><th>First Name Middle Name</th><th>Last Name</th></tr>n");
foreach ($students as $key=>$value){
// after every five records close the table and start a new one
if (($key % 5 == 0)&& ($key != 0)) {
print("</table>n<br />");
print("<table border=1><col width="50" /><col width="200" /><col width="150" /><tr><th>CWID</th><th>First Name Middle Name</th><th>Last Name</th></tr>n");
}
print_row($students[$key]);
}
print("</table>");
?>
[/sourcecode]
See my other PHP code tutorials here :
All posts tagged with “PHP”
In this program line no 32 is make a error(syntax error unexpected T_LNUMBER) this error is require so plz help me to solve this program….