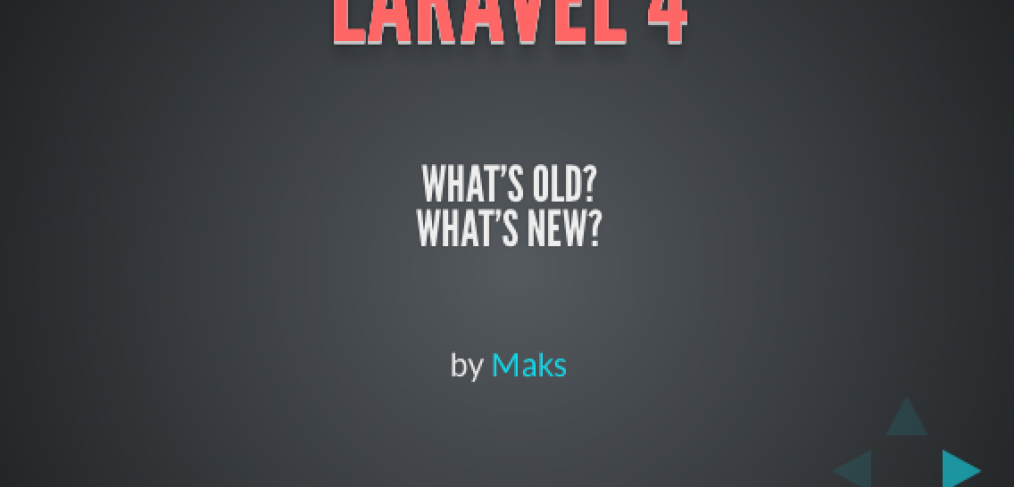
What’s new in Laravel 4
I created this presentation for a Laravel Meetup that I organized.
It explains what’s changed in Laravel v4 over v3 and brings up some nice examples of using new features.
Check it out :
And the transcript of it is below:
laravel 4
What’s old?
What’s new?
Old:
- Clean and Eloquent syntax.
- Separation of elements
- Awesome community
- Joy to code with
- Same Creator and visionary – Taylor
- Still PHP, but better (like traits in PHP 5.4)
New:
- Command line is a lot more powerful
- Testability (all components Unit Tested)
- Mail component built in
- Packages instead of Bundles (7000 vs 200)
- PHP >= 5.3.7
- Resourceful routes + controllers
- PSR-0 camelCase instead of snake_case
Command line magic
php artisan controller:make PhotoController
Produces RESTful controller:
<?php class PhotoController extends BaseController { public function index(){} public function create(){} public function store(){} public function show($id){} public function edit($id){} public function update($id){} public function destroy($id){} }
In Routes.php :
Route::resource('photo', 'PhotoController');
Command Line Magic
More Artisan commands
Make your own commands
php artisan command:make FooCommand
Testing and TDD
$response = $this->call('GET', 'user/profile');
DOM crawler:
$this->assertCount(1, $crawler->filter('h1:contains("Hello World!")'));
Packages :
- MongoDB
- LessPHP
- Bootstrapper
- Former
- Coffee – coffescript compiler
- Flatten
- 6994 more packages.
Mail::send('emails.welcome', $data, function($m) { $m->to('maks@example.com', 'Maks')->subject('Welcome!'); });
Title
Gone : STR:: and HTML:: helpers
Different Routing
Route::get('user/{name?}', function($name = 'John')
{
return $name;
});
Route::get('user/{name}', function($name) { // }) ->where('name', '[A-Za-z]+');
Route::get('user/{id}', function($id) { // }) ->where('id', '[0-9]+');
Sub domain Routing
Route::group(array('domain' => '{account}.myapp.com'), function()
{
Route::get('user/{id}', function($account, $id)
{
//
});
});
Route prefixing
Route::group(array('prefix' => 'admin'), function()
{
Route::get('user', function()
{
//
});
});
Well its looking nice than the previous obviously previous version was great but this one is too much extra best !
Hats off !