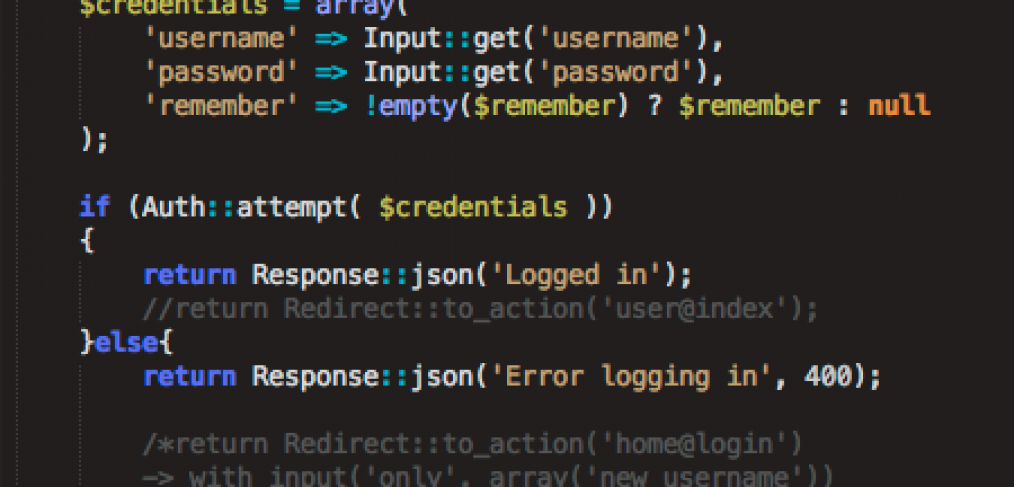
Login to Laravel web application from PhoneGap or Backbone
Login with AJAX – including PhoneGap apps
Oh God. For a few weeks I was researching what is a good way to login to a Laravel web app via AJAX and it turns out I was over-preparing for some problems that after all weren't even there.
Example – you made a web app in Laravel and you want users to be able to login via AJAX call – including a Backbone (or whatever JS framework) and PhoneGap based application.
At first I thought Laravel wouldn't pass the session to the client, or that there would be some sort of problem with that.
Turns out you don't have to overthink it and just do it like you would with any other HTML view – based application, just change things slightly to return some sort of status to your client side application. Cannot be easier to implement.
Here is an example.
You need your Laravel application to use Auth, so configure that first, and create a test user somehow (I make a simple controller that on GET will create a new user and hash a password and save the user).
In your routes.php you simply take a POST request from your form (that form will eventually live on the client side – backbone and/or PhoneGap) and direct it to HTTPS (secure route, I guess you need SSL enabled and configured in Laravel and on your hosting first):
Route::secure('POST', 'login', function()
{
$remember = Input::get('remember');
$credentials = array(
'username' => Input::get('username'),
'password' => Input::get('password'),
'remember' => !empty($remember) ? $remember : null
);
if (Auth::attempt( $credentials ))
{
return Response::json('Logged in');
//return Redirect::to_action('user@index'); you'd use this if it's not AJAX request
}else{
return Response::json('Error logging in', 400);
/*return Redirect::to_action('home@login')
-> with_input('only', array('new_username'))
-> with('login_errors', true);*/
}
});
And here is a form and jQuery that I have in my HTML form that lives on the same domain with the Laravel API (I use this to test the app before I package it in PhoneGap) :
<form class="form-vertical" id="login" method="POST" action="https://yourapp.com/login" accept-charset="UTF-8">
<input required="true" type="text" name="username" id="username">
<input required="true" type="password" name="password" id="password">
<input id="remember" type="checkbox" name="remember" value="1">Remember me</label>
<input id="loginBtn" type="submit" value="Login">
</form>
<script>
$(function() {
$('#loginBtn').click(function(e){
e.preventDefault();
$.ajax({
url: 'login',
type: 'post',
dataType: 'json',
data: $('form#login').serialize(),
success: function(data) {
alert("Logged in"); // <- this would have to be your own way of showing that user is logged in
},
error: function (xhr, ajaxOptions, thrownError) {
alert(xhr.responseText); // <- same here, your own div, p, span, whatever you wish to use
}
});
return false;
});
});
</script>
So now if you try to submit this form and launch Developer Tools -> Network Activity monitor in Chrome or Safari you should be able to see your request being processed by your route and return appropriate response (logged in or not logged in).
Meanwhile the Laravel application will setup a session and send it over along with the ajax response. It will be saved just as the regular session using a static form in HTML. Even in PhoneGap app! This way you don't have to worry about managing the session and handling the session in the client. Everything is done for you.
After the user is logged in this way, you can use regular Auth in Laravel to get user's id or permissions, etc.
For example you can specify controller Auth filter and let the user access only his/her info.
Why this works in PhoneGap is because there is no Cross-domain origin policy in place for PhoneGap apps, you can point your app to use any domain and it will work just like it was living on the same server as the application. Neat, huh?
More info on how to approve specific domain for your phonegap application : http://docs.phonegap.com/en/2.0.0/guide … st%20Guide
Any questions about this?
A note about security: This is as secure as you do it in regular web application, so it is up to you what you will use to secure your apps from someone sniffing out your packets and getting credentials. I think it is secure when you use post over https but I am no expert in this (yet). So read more about security here:
https://github.com/phonegap/phonegap/wi … m-Security
https://www.owasp.org/index.php/Top_10_ … Protection
Hey Maks,
Thanks for this post. I was overthinking such a login system as well for an upcoming Phonegap app i’m going to make. It turns out to be a lot easier!
What do you think about the Sentry package from Cartalyst? Would it be as easy to login somebody via this same approach?
Cheers
I am glad this helps! I was also doing the same mistake of overthinking this.
Why do you need to use Sentry? What is the advantage of using it?
I think the group and group permissions are a nice feature but i don’t know if i will eventually use it in the app..
Thanks for this. Helped a lot.
Is there a way where I can do this for facebook login too?
I too had been over thinking this!.
Thanks maks!
Hi, what if this form *was* on a separate domain, and what if each request was creating a separate session with Laravel? This is the problem I have… solving that is proving to be way harder.
Yeah for that you’d need a bit more ingenuity unfortunately… Have you checked out other tutorials online about this?
What about Laravel 4. It changes the rules.
having similar issue. I am able to log-in from a different domain (even local host) but the session will not persist across domains.
Please note that this will work on different domains. If you use Phonegap – it will work because Phonegap makes some exceptions about cross domain stuff and it could even store cookies across domains. Regular browser would not allow you to host a website at http://mysite.com and login/store cookies via ajax while being on http://myothersite.com. For that you would need to use much more complicated authentication mechanism, token-based authentication. The example given in this post is what’s called “session-based authentication” and it only works on the same domain or in PhoneGap.
Can you please elaborate on that a bit?