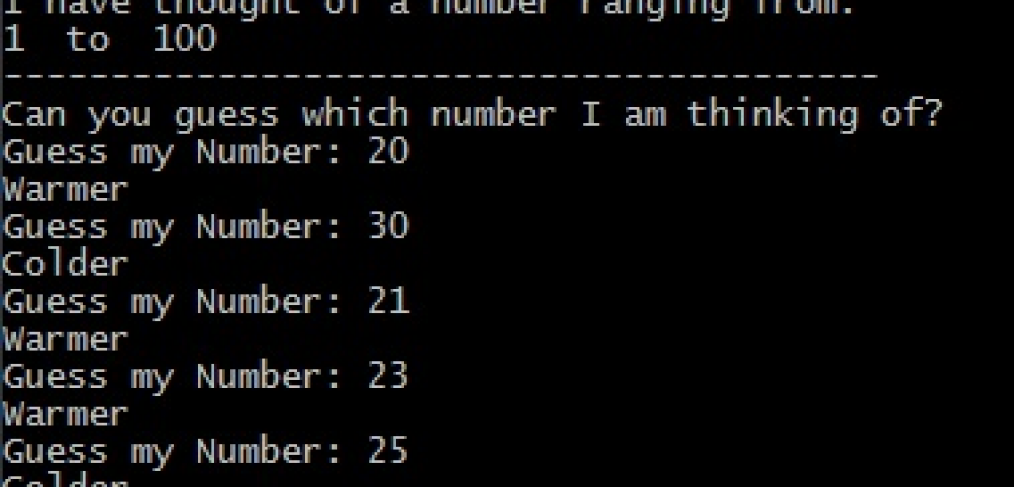
MaxoGuesso – Python game
Maxoguesso is a “Guess the number” game written in Python and ran from a command line, it was created as an assignment for Game Design class.
How to use the program:
from command line run:
guess.py [-h] [-v] [-s seed] -m 0 -M 400
Where
-h, -v, -s are optional
-s seed – Takes an int as a parameter and is used to seed the random number generator, when omitted the program, the program uses its own logic to seed the generator, for example using the current time in seconds
– v turn on debugging messages
– h print out a help message which tells the user how to run the program and brief description of the program
– m the minimum of the range of numbers the program will selects its numbers from (integer)
– M the maximum of the range of numbers the program will selects its numbers from(integer)
The program will ask the user to input her guess, then depending on user’s guess the program will respond in one of the three ways:
Warmer (meaning that user has to choose a larger number)
Colder (meaning that user has to choose a smaller number)
Winner ( correct guess)
Then the program asks the user to continue the game (start over, with a different number) or quit the game
Upon quitting, the game shows the statistics about the game played such as time played, how many guesses attempted on each game and the average number of guesses
Source code of the game :
Main file – guess.py
[python]
#==============================================================================
#Maksim Surguy
#
#Assignment 1 – Guessing Game CPSC 386 – Spring 2011
#Due : Feb 4, 2011
#
# Description:
# The main file for the Number Guessing Game, imports the MyRNG class,
# generates a random number, prompts the user to enter a guess, then compares
# user’s input to that random number, depending on how close the user is to
# the random number displays "Warmer", "Colder", "Winner !", then prompts for
# a choice to play again. Upon exiting shows statistics and time played.
#
# External Dependencies:
# math, datetime, time, sys, getopt, MyRNG
#
#
#Bugs/Features:
# bug #1 – when MyRNG is called for the first time, it returns strange float
# point numbers, overcome the bug by skipping the first call to MyRNG
#
# Features statistics about games played and time played by the user
#
#
# Parameters:
# guess.py [-h] [-v] [-s seed] -m 0 -M 400
# -h, -v, -s are optional
# -s seed – Takes an int as a parameter and is used to seed the random number generator, when omitted the program, the program uses its own logic to seed the generator, for example using the current time in seconds
# – v turn on debugging messages
# – h print out a help message which tells the user how to run the program and brief description of the program
# – m minimum Set the minimum of the range of numbers the program will selects its numbers from.
# – M maximum Set the maximum of the range of numbers the program will selects its numbers from.
#
#
#==============================================================================
import math
import datetime
import time
import sys
import getopt
from MyRNG import *
#initialize RNG with a seed of 457
r = MyRNG(457)
r.nextnumber() #due to a bug1 need to eliminate first random number
# genrandinrange asks MyRNG for a random number, then
# via simple math puts the random number in the specified range
#
# returns a random integer in the range [start…end]
def getrandinrange(start, end):
tmpnum = int(start + ((end – start) * r.nextnumber()))
return tmpnum
# setseedfromtime overwrites the seed of the MyRNG with the current second and does
# one call to the nextnumber because of the bug #1
def setseedfromtime():
currsecond = datetime.datetime.now()
r.setseed(currsecond.second)
r.nextnumber()
# gameloop prompts the user for input of a number then according to input
# tells how far the user is from the number guessed by computer
# returns the number of tries by the user
def gameloop(minim,maxim,v):
randnum = getrandinrange(minim, maxim)
if v:
print "The number guessed by computer is ",randnum
x=0
tries = 1
while randnum!=x:
try:
x = int(raw_input("Guess my Number: "))
if x<randnum :="" tries="tries" 1="" print="" warmer="" if="" x="">randnum :
tries = tries + 1
print ("Colder")
except ValueError:
print ‘Invalid Number’
print "Winner ! You Guessed in ", repr(tries)," tries"
return tries
# usage prints out a little help file
def usage( status, msg = "" ):
if msg:
print msg
print "Welcome to MaxoGuesso game !nn"
print "In MaxoGuesso, the computer generates a random number"
print "and you have to guess it in as few tries as possible"
print " "
print "To use the game, follow this format :"
print " "
print "guess.py [-h] [-v] [-s seed] -m 0 -M 400"
print " parameters -m and -M are mandatory and the integers next to them"
print " mean minimum and maximum respectively"
print ""
print " [-h] [-v] [-s seed] are optional parameters"
print " [-h] prints this file"
print " [-v] turns on verbose mode that allows to see what number"
print " is guessed by computer"
sys.exit( status )
def main( ):
setseedfromtime() # set the seed from current seconds
counters = [] #list stores how many guesses were taken to complete each game
minimum = 1
maximum = 100
verbose = False
start = time.clock()
try:
opts, args = getopt.getopt( sys.argv[1:], "hvs:m:M:" )
except getopt.GetoptError, msg:
usage( 1, msg )
for o, a in opts:
# o has the option, and a has the argument.
if o == "-h":
# Print out the usage message and exit.
usage( 0 )
elif o == "-v":
# Set verbose mode to be true for debugging messages
verbose = True
elif o == "-s":
# set the seed here
r.setseed(int(a))
elif o == "-m":
# set the minimum here (change the code below to suit your needs)
minimum = int(a)
elif o == "-M":
# set the maximum here (change the code below to suit your needs)
maximum = int(a)
if verbose:
print "Verbose mode is turned on."
print ‘*****************************************’
print ‘Welcome to MaxoGuesso game!nn’
print ‘I have thought of a number ranging from: ‘
print repr(minimum), ‘ to ‘,repr(maximum)
print ‘—————————————–‘
print ‘Can you guess which number I am thinking of? ‘
play = ‘Y’
while play == ‘Y’:
counters.append(gameloop(minimum,maximum,verbose))
play = raw_input("Want to play again? Y/N : ")
print "Game Over!nn"
# Suggested Improvements (shows statistics, time played)
print "Statistics :"
avg = 0
print "Games played: ", len(counters)
for index, item in enumerate(counters):
print ‘game ‘ , index, ‘tries’, item
avg = avg + item
print "Average tries : ", avg/len(counters)
print "Time played :" , int((time.clock() – start)), ‘seconds’
if __name__ == "__main__":
main()
[/python]
Random Number Generator Class (required by guess.py)
MyRNG.py
[python]
#==============================================================================
#Maksim Surguy
#
#
#Assignment 1 – Guessing Game CPSC 386 – Spring 2011
#Due : Feb 4, 2011
#
#Description:
# MyRNG Class generates a random number according to Lehmer’s algorithm outlined by Stephen Park and Keith Miller in
# "Random Generators: good ones are hard to find" p .1195
#
# External Dependencies:
# none
#
#==============================================================================
class MyRNG:
# initialize the class by assigning some fixed numbers to variables
def __init__(self, myseed=None) :
self.seed = myseed
self.a = 16807
self.m = 2147483647
self.q = 127773
self.r = 2836
self.tmpran = 1.0
# setseed sets the seed to a passed parameter
def setseed(self, s=None) :
self.seed = s
# nextnumber does the testing of the seed according to Park and Miller algorithm outlined on page 1195
# this testing makes sure that the numbers will be more or less random and periodic
# returns a random float number like 0.98764535876
def nextnumber(self) :
hi = (self.seed/self.q)
lo = (self.seed%self.q)
test = ((self.a*lo)-(self.r*hi))
if test > 0 :
self.seed = test
else :
self.seed = (test+self.m)
self.tmpran = (1.0*self.seed / self.m)
return self.tmpran
[/python]
You can download the whole game zipped up here: