Laravel: my first framework. Chapter 1 – Meeting Laravel
The following content is the first chapter of my new e-book titled “Laravel: my first framework“. This writing is copyright by Maksim Surguy. Unauthorized copy and distribution of this material is prohibited.
Chapter 1. Meeting Laravel
This chapter covers:
- An introduction to Laravel 4 PHP framework
- History of Laravel framework
- Advantages of using Laravel
Developing a web application from scratch can be a tedious and complicated task. Over time, too many opportunities for bugs and too many revisions can make maintenance of a web application very frustrating for any developer. Because of this, a recently developed framework written in PHP, Laravel, has quickly won the hearts of developers around the world for its clean code and ease of use.
Laravel is a free PHP framework that transforms creation of web applications into a fun and enjoyable process by providing expressive and eloquent code syntax that you can use to build fast, stable, easy to maintain and easy to extend web applications. Laravel consists of many components commonly needed in the process of building web applications. PHP developers use Laravel for a broad range of web applications, from simple blogs and CMSs to online stores and large-scale business applications. It’s no wonder Laravel has become a strong contender in the family of package-based web frameworks and is ready for prime time after only a few years of development.
In this chapter you will meet Laravel PHP framework, you will learn about its short but interesting history and its evolution over its lifetime, how by using established conventions and approaches Laravel can speed up development significantly and find out why you, too, will find Laravel a great choice for your applications. Are you ready? Let’s meet Laravel!
1.1 Introducing Laravel 4 PHP framework
Laravel 4 is an open source PHP web application framework created by Taylor Otwell and maintained by him and a community of core developers. For over two and a half years web developers around the world have been using Laravel (version 1 to version 4) to build stable and maintainable web applications by using Laravel’s powerful features such as built-in authentication, session management, database wrapper and more. Laravel is considered to be a full stack web development framework, meaning it can handle every aspect of a web application architecture – from storing and managing data using the database wrapper to displaying user interfaces using its own templating engine. Majority of the mundane work that a web developer encounters in the process of creating a web application has been thought of and alleviated by Laravel’s components. Because of this, an application can be built a lot faster than it would be if the developer made the whole application from scratch.
PHP Framework
A PHP Web application framework is a set of classes, libraries or components written in PHP server-side scripting language that aim to solve common web development problems and promote code reuse. Such components as authentication, session management, caching, routing, database operations wrappers and more are included in Laravel and most popular PHP web application frameworks. Using a web application framework allows developers to save significant amount of time developing a web application.
While being a great time saver, Laravel isn’t a magic cure for all your web development problems. Although it provides a solid foundation for the backend of your application, you still need to come up with the application logic, database structure and the HTML, CSS and Javascript for you application. Also, Laravel (and PHP scripting language in general) isn’t well suited for real time applications like web chats, video streaming, real time gaming, and instant messaging so you would have to use something else for those kinds of applications.
The architecture of Laravel makes it fitting for building many kinds of web applications. From simple blogs, guestbooks, and single-purpose websites to complex CMSs, forums, APIs, e-Commerce websites and even social networks, Laravel will help you get web application development done faster.
It’s not just the features that make Laravel so special. There are many reasons to use Laravel in your next web development project. Web developers choose Laravel for the expressive and clean code that it provides as a basis for web applications. The expressiveness is what distinguishes Laravel from other similar PHP frameworks. The application structure that Laravel provides makes it easy to start working even for a beginner developer that has never used a framework before. The community around Laravel, including the creator of the framework himself, has been very welcoming and supportive. Let’s explore these reasons a bit more below. How does Laravel’s expressive code help you, the developer, to keep the code of your application maintainable, easy to change and easy to read?
1.1.1 Laravel’s Expressive code
The most prominent candidates for code re-use in web development with PHP are managing sessions, authentication and operating with data stored in databases. Laravel has tackled those areas quite gracefully and offers beautiful syntax to help you develop applications. Listing 1.1 shows a handful of examples of Laravel’s expressive syntax:
Listing 1.1 Examples of Laravel’s expressive syntax
$order = array('customer' => 'Prince Caspian', 'product' => 'Laravel: my first framework');
// Store the order details in the session under name "order"
Session::put('order',$order);
...
// Retrieve the order details from the session using the session element called "order"
// and create a new record in the database
$orderInDB = Order::create(Session::get('order'));
// Remove the session element "order"
Session::forget('order');
// Retrieve all orders for customer "Prince Caspian"
$ordersForCaspian = Order::where('customer', 'Prince Caspian')->get();
...
// Count all orders of "Laravel: my first framework"
$ordersQuantity = Order::where('product', 'Laravel: my first framework')->count();
Laravel promotes usage of clean and expressive code throughout your web application while it still remains PHP code. All of Laravel’s method names are logical, sometimes to an extent that you can even guess them without looking up the documentation. For example to detect if there is an item called ‘order’ in the session already you would use Session::has('order')
which might come naturally to your mind. The same could be said about the sensible defaults for all methods that need to return some value: session items form input elements, cache items, and more. As another minimal example, you could return a default value for an input submitted with a form if nothing was provided by the user: Input::get('name', 'Guest Customer')
.
These conventions might not immediately appeal to every developer, but in the long run they make the framework consistent and unified. Laravel makes many assumptions that accelerate web development tremendously. While developing applications with Laravel you will enjoy those moments when the framework appears to know exactly what you intend to do. Aside from the expressive and clean code, Laravel makes your applications easier to maintain by adopting separation of the application code into files that work with the data, files that control the flow of the application and files that deal with the output that the end user will see.
1.1.2 Laravel applications use Model-View-Controller pattern
When you install Laravel you will notice that inside of the “app” folder there are folders called “models”, “views”, “controllers” amongst other folders. This is because Laravel is a framework for MVC applications. Such applications use the Model-View-Controller software architecture pattern, which enforces separation between the information (model), user’s interaction with information (controller) and visual representation of information (view). As you develop your Laravel applications you will mainly work with files inside of those three folders. Don’t worry if you are not familiar with MVC pattern yet, Laravel is a great framework to learn MVC and there is a section of this book that will give you an introduction to this software pattern and its usage in Laravel applications.
Consider having your whole web application in one PHP script. Mixing SQL statements, business logic and output all inside one file will be very confusing for any developer to work on. Especially if it has been quite some time since you have looked at the code of such application. As the application grows it will become harder or impossible to maintain. The main benefit of using MVC architecture in Laravel applications is that it helps you have the code of your application separated and organized in many small parts that are easier to change and extend. You will come to appreciate this separation when you will want to add a new feature into your application or when you will need to quickly modify existing functionality. Laravel has been around for more than two years. Even though that doesn’t seem like a lot, it has matured and has been road tested by thousands of developers worldwide. The developers that use Laravel communicate to each other through many different online and offline channels, sharing tips, code examples, and helping maintain the framework.
1.1.3 Laravel was built by a great community
Contrary to popular belief, Laravel is not one-man’s product. While Taylor Otwell is the main developer behind the framework – the visionary and the person in charge of saying “yes” or “no” to features, the open source nature of Laravel made it possible to get code contributions and bug fixes from over a hundred developers worldwide, Laravel’s public forums (with over 20,000 registered users) and a thriving IRC channel helped steer the development and testing of the framework. Laravel’s vibrant and welcoming community at http://laravel.com is something that makes Laravel special and distinctive from other similar projects.
Laravel would not be in the place where it is now if not for contributions, ideas, suggestions and passion of so many developers and lovers of clean code all around the world. In fact, many Laravel features were inspired by other open source projects like Sinatra, Ruby on Rails, CodeIgniter and others. How did Laravel come such a long way in just over two years? Let’s explore Laravel’s history and see how it evolved over time.
1.2 History of Laravel framework
Rome wasn’t built in a day, and so weren’t any of the established frameworks. Usually building a solid web development framework that is well tested and ready for production deployment takes a long time. Though interestingly, in case with Laravel its development was progressing a bit differently and at a faster pace than other frameworks.
1.2.1 State of PHP frameworks world before Laravel 4
In August of 2009 PHP 5.3 was released. It featured introduction of namespaces and anonymous functions called closures amongst other updates. The new features allowed developers write better Object-Oriented PHP applications. Even though it provided many benefits and a way to get to a brighter development future, not all frameworks were looking into that future but instead focused on supporting the older versions of PHP. The framework landscape mainly consisted of Symfony, Zend, Slim micro framework, Kohana, Lithium and CodeIgniter.
CodeIgniter was probably the most well known PHP framework at the time. Developers preferred it for its comprehensive documentation and simplicity. Any PHP programmer could quickly start making applications with it. It had large community and great support from its creators. But back in 2011 CodeIgniter was lacking some functionality that Taylor Otwell, the creator of Laravel, considered to be essential in building web applications. For example out of the box authentication (logging users in and out) and closure routing were absent in CodeIgniter. Therefore, Laravel version 1 beta was released on June 9, 2011 to fill in the missing functionality. According to Laravel’s creator, Taylor Otwell, Laravel version 1 was released in June 2011 simply to solve the growing pains of using CodeIgniter PHP framework.
1.2.2 Evolution of Laravel framework
Starting with the first release, Laravel featured built-in authentication, Eloquent ORM for database operations, localization, models and relationships, simple routing mechanism, caching, sessions, views, extendibility through modules and libraries, form and HTML helpers and more. Even on the very first release the framework already had some impressive functionality. Laravel went from version 1 to version 2 in less than six months.
The second major release of the framework got some solid upgrades from the creator and the community. Such features were implemented: controller support, “Blade” templating engine, usage of inversion of control container, replacement of modules with “bundles” and more. With the additions of controllers, the framework became a fully qualified MVC framework. Less than two months later new major point release was announced, Laravel 3.
The third release was focused on unit test integration, the Artisan command line interface, database migrations, events, extended session drivers and database drivers, integration for “bundles” and more. Laravel 3 was quickly catching up to the big boys of PHP frameworks such as CodeIgniter and Kohana, a lot of developers started switching from other frameworks to Laravel for its power and expressiveness. Laravel 3 stayed in a stable release for quite some time but about 5 months after it was released the creator of the framework decided to re-write the whole framework from scratch as a set of packages distributed through “Composer” PHP dependency manager. Thus Laravel 4 codenamed “Illuminate” was in the works.
Laravel 4 was re-written from the ground up as a collection of components (or packages) that integrate with each other to make up a framework. The management of these components is done through the best PHP dependency manager available called “Composer”. Laravel 4 has an extended set of features that no other version of Laravel (and even no other PHP framework) has had before: database seeding, message queues, built in mailer, even more powerful Eloquent ORM featuring scopes, soft deletes and more.
Embracing the new features of PHP 5.3 Laravel has come a long way in just over two years appealing to more and more developers worldwide. The visionary behind the framework – Taylor Otwell and the community surrounding Laravel have made enormous progress creating a future-friendly established architecture for PHP web applications in a very short period of time. Holding this book testifies on Laravel’s success and constantly growing community of users and contributors means that Laravel is here to stay. But what makes Laravel so beneficial for developers, and why should you care to use it in your projects instead of hand made code or instead of other available frameworks?
1.3 Advantages of Using Laravel
There are many advantages to using Laravel instead of hand-made code or even instead of other similar frameworks. Laravel is a winner because it incorporates established and proven web development patterns: convention over configuration so it is ready for use out of the box, Model-View-Controller (MVC) application structure and Active Record powering its database wrapper. By using these conventions and patterns Laravel helps you as a developer to build a maintainable web application with easy to understand code separation and in a short time frame.
Without a framework like Laravel maintenance or adding new features to a web application could become a nightmare. Lack of structure will create problems when another developer will want to fix bugs or extend the application. Absence of standards will make it hard to understand application’s functionality even for most experienced developer. Unconventional method and function names might make the code of the application unreadable and low quality. Laravel helps you avoid all of these problems.
Using commonly accepted conventions and established software development patterns Laravel helps your applications stay alive and relevant. Over the next few pages I will take you on a tour exploring the advantages of using Laravel framework over hand made code or other frameworks. First destination: “Convention over configuration”.
1.3.1 Convention over configuration
Imagine if you could do a fresh install of the framework and in the next five minutes already be working on how your application behaves when a user visits a certain page and not worry about configuring the things that are common to most applications? Laravel makes many assumptions that make this kind of scenario possible, this is known as “convention over configuration” pattern. When you start writing a new web application you would like to have as minimal initial set up as possible. Such configuration settings as the location where the application will save your sessions and cache, what database provider and what tables in the database it will use, locale of the application and more settings are usually needed for web applications. Thankfully all these configurations are already pre-set for you in a fresh installation of Laravel, but, if needed, any changes to these configurations could be easily made.
Convention over configuration
Convention over configuration is a software design pattern that minimizes the number of decisions that a developer needs to make by implying reasonable configuration defaults (conventions) requiring the developer to provide the configuration for only those parts that deviate from the conventions.
The configuration of new Laravel applications is very minimal and it is all separated for you inside of one folder: “app/config”. You need to only change the settings that are different from pre-set conventions. For example provide a database name if you are using one, your time zone, and you are good to go. If you are not using a database or don’t care about time zone settings, then you don’t even have to do any configuration and can start coding your application right away! All the other settings are set to reasonable defaults and you need to change them only if your application departs from pre-set conventions. We will explore Laravel’s configuration more in detail in the next chapter. This smart way of minimizing the amount of decisions that a developer has to make to start developing an application makes Laravel very easy to get started with and appealing to developers of all proficiency levels.
1.3.2 Ready out of the box
With some frameworks it could take hours just to get to a point where the developer can start using the framework. Laravel is unique in this sense because you can install and start using Laravel in just few minutes. When you install Laravel for the first time you will notice that you don’t need to do anything else to have your application operational. After starting a server using built in command you can right away visit the root URL of the application and get a response from Laravel signifying that your Laravel application is ready (figure 1.1):
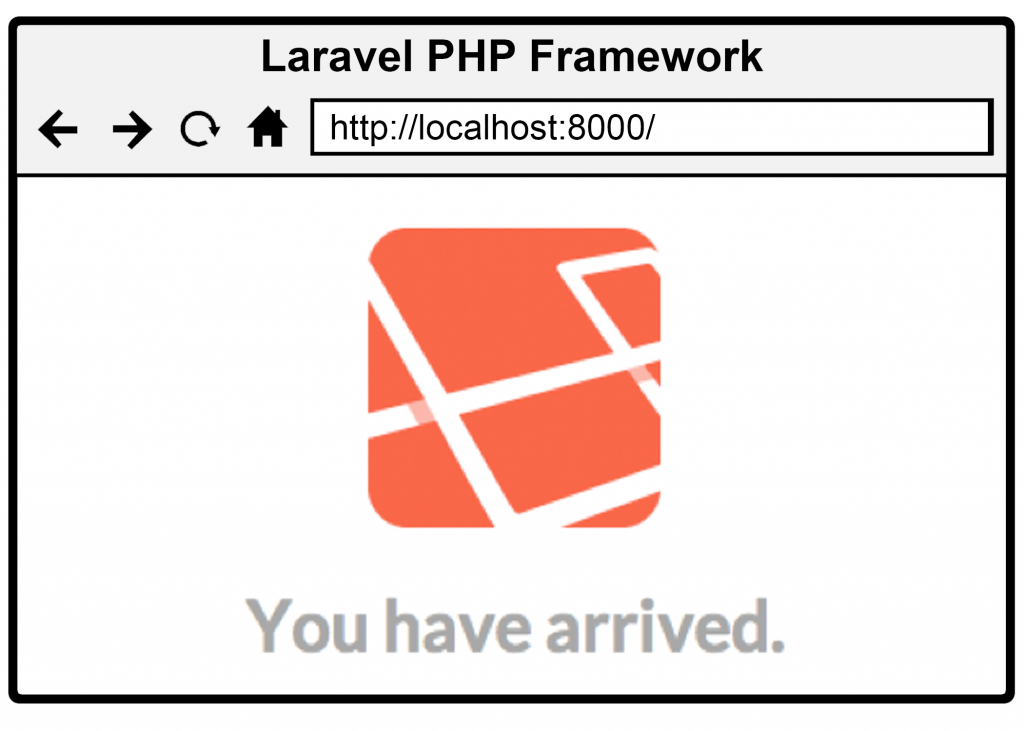
Figure 1.1 Laravel is operational out of the box with zero configuration
Amazingly when this page shows up Laravel already assumes that you are developing locally, it knows where to save the cached pages, it knows that going to the application’s root URL is supposed to display a page with some pre-set content in it. This is great because you can get to the development of your application’s logic, models and responses in no time. Not only your application is ready for your coding attention, it already has some great structure to it.
1.3.3 Clear organization of all parts of the application
Everything has its own place in a Laravel application. Because applications built with Laravel follow MVC pattern, the data models live in app/models
folder, the views reside in app/views
folder. Can you guess where the controllers are? You got it right, in app/controllers
folder! This separation makes your code cleaner and easier to find. For example if you have a database table called “orders” you will most likely have a model for it called “order” in your models folder. If you have created a nice login screen for your application it will be somewhere in the views folder. Take a look at figure 1.2 to see which folders your Laravel applications will consist of.
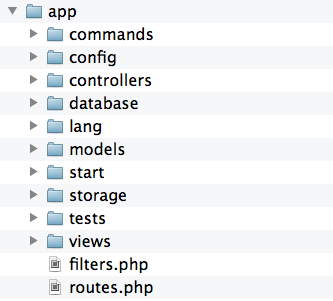
Figure 1.2 Folder structure of a Laravel application
There are a few more folders other than models, views and controllers but don’t be afraid, there will be a whole section of the next chapter devoted to all of these folders because together they are the heart and mind of your applications (while Laravel itself is the soul). Each file and folder has its purpose but by conventions they are already pre-filled with something so you can get started quicker and change only the parts that need to break out of the conventions. There are many things that made Laravel so successful. Clean organization is certainly one of those things. Another one is built-in Authentication.
1.3.4 Built-in Authentication
An important feature that has been missing in some other big name frameworks is built-in authentication for web applications.
Authentication
Authentication is the process of identifying a user, usually by some unique user attribute (username or email) and accompanying password, in order to grant access to some specific information.
Since its first release, Laravel featured authentication methods to log users in and log them out and also making it easy for a developer to access properties of the currently logged-in user. Laravel 4 only continues the trend of authentication being pre-baked into the framework and greatly simplifies the process of authentication. In listing 1.2 we will explore some authentication methods that Laravel provides. By default authentication uses a table called “users” in your database and the user model is already in the app/models folder. Please note that the following code is just to demonstrate the simplicity of authentication methods so it is not a part of any particular file (we will get to that in detail later):
Listing 1.2 Demonstration of Laravel’s built-in authentication methods
// Provide username and password to check against (could be from input)
$user = array('username' => 'caspian356', 'password' => 'P@s5w0rD');
// Check user's credentials against user's details in the DB table called "users" and log the user in
if (Auth::attempt($user))
{
return 'User has been logged in successfully';
}
else
{
return 'Login failed';
}
...
// Check if the user's browser has a cookie matching Laravel's session contents
if (Auth::check())
{
return 'User is logged in';
}
...
// Retrieve some attributes of the currently logged in user
$username = Auth::user()->username;
echo $username;
// Outputs 'caspian356'
...
// Log the user out, by erasing the login cookie from the browser and destroying user's session
Auth::logout();
These are just a few examples of Laravel’s incredible authentication functionality. The list above is not a comprehensive list of all authentication methods Laravel 4 has up its sleeve. There are also password resets through a link sent in the email, HTTP Basic authentication (great for APIs) and of course built in methods of protecting areas of your web application. In later chapters we will get to know them better but for now let’s take a look at another reason why Laravel is a great framework to use for your next web development project – methods of working with contents of databases.
1.3.5 Eloquent ORM – Laravel’s Active Record implementation
Working with data is an essential part of all web applications. Usually PHP web applications store data in rows of database tables. Starting with version 5.0 the PHP language introduced a unified interface to query databases and to fetch the results. While those new features were helpful and enough for some basic applications, they made application’s code look like a mess of SQL statements interwoven with PHP code. Also they did not add much convenience to working with data that is related with other data by means of foreign keys. To alleviate that imagine if you could present the data stored in a database table as a class with each row being an object. That would simplify access to the data in database and also would make it possible to relate the data by means of assigning objects to other objects’ properties. This technique of wrapping DB data into objects is called Active Record pattern. Laravel implements Active Record pattern in its “Eloquent ORM”.
ORM
Object Relational Mapping (ORM) enables access to and manipulation of data within a database as though it was a PHP object.
Laravel’s Eloquent ORM allows developers to use Active Record pattern in full extent making application’s code that deal with database look like clean PHP and not like messy SQL. Creation of new entries in database, manipulating and deleting entries, querying the database using a range of different parameters and much more are made easy and readable with Eloquent ORM. Eloquent makes quite a few assumptions about your databases but at the same time all of those assumptions could be overridden and customized (again, convention over configuration). For example it expects the primary key of each table to be called “id” and it expects the name of the tables to be plural of the model’s name.
Let’s say you have a table called “shops” in a database. A model that corresponds to that table would have to be called “Shop”. If you wanted to use Eloquent ORM to create a new row in “shops” table containing the details of a new shop, you would do that by creating a new object of class Shop and operating on that object. This is done with code in listing 1.3:
Listing 1.3 Demonstration of Eloquent ORM creating a row in database
// Define the Eloquent model for "shop" in app/models/shop.php file
class Shop extends Eloquent {}
...
// Create a new instance of the model "shop"
$shop = new Shop;
// Assign content to the columns of the database row that will be created
$shop->name = 'Best Coffee Co';
$shop->city = 'Seattle';
// Perform a save of the new row into the database
$shop->save();
With Eloquent ORM you are not limited to operating on just one table in the database. Built-in relationships allow you to manipulate related models and in turn related tables. Eloquent assumes that you have a foreign key set up in format “model_id” on the tables that need to be related to a particular model. For example if you have a table called “customers” containing foreign key called “shop_id” and wanted to relate the newly created shop to a new customer, you would use code that follows in listing 1.4:
Listing 1.4 Using Eloquent relationship to create related model
// Define Eloquent model for "customer" in app/models/customer.php file
class Customer extends Eloquent {}
...
// Eloquent model of "shop"
class Shop extends Eloquent {
// One-to-many Eloquent relationship that relates model "shop" to model "customer"
public function customers()
{
return $this->hasMany('Customer');
}
}
...
// Create new object of "customer" Eloquent model
$customer = new Customer(array('name' => 'Prince Caspian'));
// Create a new row in table "customers" while setting "shop_id" column
// to the "id" of the shop that was created previously
$customer = $shop->customers()->save($customer);
The examples above are just a tiny tip of the iceberg of what’s possible with Eloquent. As you can see, Eloquent makes manipulating database data and related data a breeze while keeping the code of the application clean and easy to read. This book offers a whole chapter devoted to this powerful and unique ORM because being able to work with it will save you countless amounts of time. As many other developers did, you will find it a joy working with Laravel’s Eloquent ORM.
1.4 Summary
You have been introduced to Laravel PHP framework and have learned about its history and importance. Laravel has matured very quickly in just over two years. While being a young and fresh framework, it uses well established software design patterns:
- MVC application structure to keep the code separated while maintaining flexibility
- Active Record in its Eloquent ORM to simplify working with data inside of a database
- Convention over configuration to keep the setup minimal
You have learned that Laravel is a full stack framework. It features built in authentication, it has had a unique and powerful ORM since the first release, it is ready to be used out of the box with minimal configuration. From templating to working with data, Laravel has you covered. Applications built with Laravel feature code that is both expressive and clean at the same time, which makes them easier to maintain.
In the chapters that follow you will become a Laravel expert. Let’s begin this journey by installing Laravel and making our first application with it!
Just had surgery and I’m off my feet for a while. I’m a WordPress developer that has been playing with Laravel for just over a year with a lot of half finished projects.
Your book has given me the push to actually take a project from start to finish. The level and way you explain the different parts have resonated with me and I really appreciate it.
I’ll definitely let you know when the project is online and making me money!
Thanks again.
Eric
Thanks for the feedback!
I’m glad the book is helpful and is persuading enough to take up this great framework 🙂
Feel free to get the PDF version on Leanpub if you want a more convenient format of reading.
Thanks for this tutorial. Good way to start off.