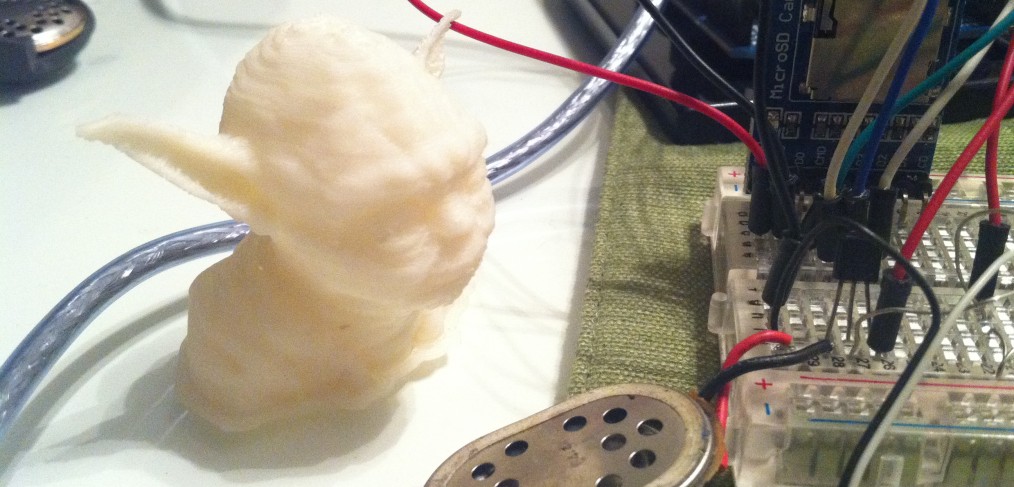
How to play WAV audio files with Arduino Uno and MicroSD card
A couple days ago I printed Yoda on my 3d Printer – http://instagram.com/p/U2HR__StrH/
Yesterday I put him on a remote controlled micro robotic platform, watch video of it in action here:
And today I made him speak.
The basics setup is this :
- Arduino Uno.
- MicroSD card reader (4$ at DX.com ).
- MicroSD card (6$ on Amazon)
- Speaker and a transistor to act as amplifier.
- LED
- 3D printed Yoda.
I found some Yoda WAV sound files online and encoded them from iTunes into 8bit 16Khz mono sound. Then put the files on the microSD card from the computer.
Put the MicroSD card into the card reader.
Hook up the card reader as follows:
- 3.3v goes to 3.3v on the Arduino UNO (for power)
- GND goes to Ground on Arduino UNO
- D0 goes to pin 12 on Arduino UNO
- D1 goes to pin 11 on Arduino UNO
- CLK goes to pin 13 on Arduino UNO
- D3 goes to pin 10 on Arduino UNO
Now you are able to read and write files to the MicroSD card.
The next step is to download this Arduino library to play WAV files and install it :
https://github.com/TMRh20/TMRpcm
Here is the code for my Arduino sketch :
#include <SD.h> // need to include the SD library
//#define SD_ChipSelectPin 53 //example uses hardware SS pin 53 on Mega2560
#define SD_ChipSelectPin 10 //using digital pin 4 on arduino nano 328
#include <TMRpcm.h> // also need to include this library...
TMRpcm tmrpcm; // create an object for use in this sketch
char mychar;
void setup(){
tmrpcm.speakerPin = 9; //11 on Mega, 9 on Uno, Nano, etc
Serial.begin(9600);
if (!SD.begin(SD_ChipSelectPin)) { // see if the card is present and can be initialized:
Serial.println("SD fail");
return; // don't do anything more if not
}
tmrpcm.play("beware.wav"); //the sound file "music" will play each time the arduino powers up, or is reset
}
void loop(){
if(Serial.available()){
mychar = Serial.read();
if(mychar == 'o'){ //send the letter p over the serial monitor to start playback
tmrpcm.play("helpyou.wav");
} else if(mychar == 'r'){ //send the letter p over the serial monitor to start playback
tmrpcm.play("chortle.wav");
} else if(mychar == 'q'){ //send the letter p over the serial monitor to start playback
tmrpcm.play("helpyou.wav");
} else if(mychar == 'p'){
tmrpcm.play("beware.wav");
}
else if(mychar == 'w'){ //send the letter p over the serial monitor to start playback
tmrpcm.play("impresiv.wav");
}
else if(mychar == 't'){ //send the letter p over the serial monitor to start playback
tmrpcm.play("seekyoda.wav");
}
else if(mychar == 'y'){ //send the letter p over the serial monitor to start playback
tmrpcm.play("sensefear.wav");
}
else if(mychar == 'u'){ //send the letter p over the serial monitor to start playback
tmrpcm.play("strongami.wav");
}
else if(mychar == 'i'){ //send the letter p over the serial monitor to start playback
tmrpcm.play("whyhere.wav");
}
}
}
Upload that sketch to the Arduino after installing the TMRpcm WAV library.
All that’s left is connecting a speaker!
I found a spare transistor (2N2222) and hooked it up before connecting the speaker (you can also use a piezo speaker without transistor just to test if it works as intended),
Follow this schematic to connect the speaker to the arduino : http://blog.bryanduxbury.com/2012/01/one-transistor-audio-amplifier-for.html
My speaker is on pin 9 so do the same and you will be all set.
After connecting the speaker and uploading the sketch I open up serial connection terminal and send commands – letters from q to p on the keyboard, sending each character will play a different sound on the Arduino.
Now you can play audio files through your Arduino, on the cheap and with a lot of fun to come!
Here’s a shopping list for making a similar project:
I like your breadboard… and Great Job… hehe…
Is this project using the newest TMRh20 libraries with automatic detection sample rate? if i’m not wrong, it can change the volume too…
I would like to make robot sound, if any input trigger different sound, eg. input A –> sound1.wav , input B –> sound2.wav, input C –> sound3.wav, etc.
It will be helpful if you modify the sketch for us,
Thank you
NB : Sorry if my english is terrible.
Yes it is using the latest library, I haven’t tried changing the volume…
Well, to modify it all you need to do is this, instead of
if(Serial.available()){
mychar = Serial.read();
you have to put the code to play sound inside of each button “if statements” (http://arduino.cc/en/tutorial/button)
From the code in that link you will just have :
if (buttonState == HIGH) {
// play your sound:
tmrpcm.play(“sound1.wav”);
}
Does this make sense?
I’ve tried with atmega8 burned with arduino bootloader,
but failed to compile :
E:Micro ControllersArduinoarduino-1.0.1-windowsarduino-1.0.1librariesTMRpcmTMRpcm.cpp: In function ‘void __vector_5()’:
E:Micro ControllersArduinoarduino-1.0.1-windowsarduino-1.0.1librariesTMRpcmTMRpcm.cpp:125: error: ‘TIMSK1’ was not declared in this scope
E:Micro ControllersArduinoarduino-1.0.1-windowsarduino-1.0.1librariesTMRpcmTMRpcm.cpp:125: error: ‘ICIE1’ was not declared in this scope
E:Micro ControllersArduinoarduino-1.0.1-windowsarduino-1.0.1librariesTMRpcmTMRpcm.cpp: In member function ‘void TMRpcm::startPlayback()’:
E:Micro ControllersArduinoarduino-1.0.1-windowsarduino-1.0.1librariesTMRpcmTMRpcm.cpp:191: error: ‘TIMSK1’ was not declared in this scope
E:Micro ControllersArduinoarduino-1.0.1-windowsarduino-1.0.1librariesTMRpcmTMRpcm.cpp:191: error: ‘ICIE1’ was not declared in this scope
E:Micro ControllersArduinoarduino-1.0.1-windowsarduino-1.0.1librariesTMRpcmTMRpcm.cpp: In member function ‘void TMRpcm::stopPlayback()’:
E:Micro ControllersArduinoarduino-1.0.1-windowsarduino-1.0.1librariesTMRpcmTMRpcm.cpp:202: error: ‘TIMSK1’ was not declared in this scope
E:Micro ControllersArduinoarduino-1.0.1-windowsarduino-1.0.1librariesTMRpcmTMRpcm.cpp:202: error: ‘ICIE1’ was not declared in this scope
E:Micro ControllersArduinoarduino-1.0.1-windowsarduino-1.0.1librariesTMRpcmTMRpcm.cpp: In member function ‘void TMRpcm::disable()’:
E:Micro ControllersArduinoarduino-1.0.1-windowsarduino-1.0.1librariesTMRpcmTMRpcm.cpp:210: error: ‘TIMSK1’ was not declared in this scope
E:Micro ControllersArduinoarduino-1.0.1-windowsarduino-1.0.1librariesTMRpcmTMRpcm.cpp:210: error: ‘ICIE1’ was not declared in this scope
I think this script need to modify in order to use on atmega8, but i don’t know what to modify.
Oh, I think they are using different timers, don’t know if there is a way to modify it to work on Atmega8. Why can’t you use Atmega 328?
Hallo Maks I would like some help.. not really related to the project in many ways at all other than audio. I have my arduino set up to light when I get e-mails and messages.. but can’t seem to apply sound to it when I get one. I would like to use a simple note. so I was planning on using the default sound example for the audio.
Are you using piezo speaker, or something else?
Speaker specifications?
You mean the one I used?
Hello Maks,
Thanks for a great library!
I’m trying to mimik an RF remote control. I’ve recorded command sample with Audacity and saved it to 8bit unsigned Windows PCM format (*.wav).
Hardware setup as follows:
– Arduino Uno
– Ethernet Shield with microSD slot
For the unknown to me reason, Arduino plays only small part of file, then hangs.
Could you, please, help me figure out why this is happening?
Link to file which hangs Arduino with TMRpcm: http://yadi.sk/d/KbYuAeAK36D29
Link to file which I would like to play: http://yadi.sk/d/f78p9f6236D6h
I’d grateful for you help.
Can you try to export files from iTunes instead, like it is specified in the library? I think the bitrate or some encoding is incorrectly set. You can also try another converter for the sound.
Let me know if iTunes way works for you
funny, I’ve been sure, that iTunes is a total crap (no offense). Finally 84meg download is worth it.
You were right: iTunes conversion works for TMRpcm. But still there is a catch.
It does only play file once. To play it again I need to reboot Arduino. Another thing is resulting waveform. In some parts it resembles original track, but whereas original track is uninterrupted, Arduino playback has visible interrupts. And although your project is awsome, thins little thing kills my idea of transmitting RF command.
To clear things up: I’ve connected RF OOK/ASK transmitter to pin 9, and plugged in RF receiver into my notebook’s mic port to visualize waveforms in Audacity.
That is how I know that waveforms (original and playback) in some parts are almost exact match, and that there are interrupts in playback.
I will have to find another way around, but not really sure if I can.
Your program is awesome. I tried using this and succeeded in playing some of the .wav files. But some .wav files are not playing. I checked those files over their sample size(8 bit), channel(mono), bit rate(256 kbps), Sample rate(16KHz). Every thing is the same. But I do not know why some .wav files have problem in playing. Will anyone clarify me about the problem?
Thank you in Advance.
I believe the bit rate is set too high. Try 44100 Khz
help plz…
i tried all ta i cna do but everytime i compile i get..
SampleRate Too High: Wrong BitRate
what is the correct bit rate and how do i change the bit rate???
i am using arduino328
Try 44100 HZ
yeah used 44100 hz, 16 bit and mono..
and i end up in 705 kbps..
still it says SampleRate Too High: Wrong BitRate
can u brief me how to change the bit rate..
i tried changing using a software called audacity.
i now have my original wav file with bitrate 176kbps.
what are the things i should do to make the wav file play in arduino..
kindly help 🙂
First try setting the sound to 16.000kHz, 8-bit, Mono
If that doesn’t work, can you try using iTunes to convert the files? Here is the instruction how to convert with iTunes:
https://github.com/TMRh20/TMRpcm/blob/master/TMRpcm/README
Thank You so Much 🙂 its working 🙂 🙂
Awesome!!! =))) Glad to hear that!
Where did you get that speaker? Really need one for my school project. Can i just buy a regular small speaker and do something to connect the wires?
Yes, any small regular 8 Ohm speaker should be ok!
I designed the library to handle files between about 8Khz and 20Khz, other than that the files have to be 8-bit and Mono. It should play any standard WAV file in that format.
TMRh20
Hi Maks, This is just the type of project I need to finish off my transformers bumblebee costume with sounds.
I have made my own helmet but I would love the sounds of the bought helmet.
I have an arduino and I want sounds to play one after the other at the push of a button or separate buttons.
Could you tell me how to wire this up and a code to use.
Thanks.
Greg
What have you tried so far?
I want to thank TMRh20 for this library. Spectacular!
I’m making a doorbell that plays different sounds when pressed. Any suggestions on an amplification circuit so it can be heard throughout the house?
Thanks
Hi Maks,
Thanks for your reply but i worked it out.
Found a great set of instructions for this sketch…
http://www.hackerspace-ffm.de/wiki/index.php?title=Datei:SimpleSDAudio_V1.03.zip
… and used some instructions on changing the file to play on arduino from here…
http://hlt.media.mit.edu/?p=1963.
So now what I do is save a wav or mp3 file, then convert it in itunes and then save it onto a micro sd card and insert this into my arduino uno.
Then all I have to do is change one line in the sketch to play my converted wav file and upload.
The last file I converted and played was 60 seconds long and sounded great.
My sd card is only 512 meg but played it no problems.
I will try a bigger file tomorrow to see how much I can fit on this card.
The next step is to have different buttons for different wav files. Found a great set of instructions here to allow many buttons on one pin. Looks too easy but I gotta try it.
http://www.instructables.com/id/How-to-access-5-buttons-through-1-Arduino-input/?ALLSTEPS
Yaiii! good job!
Let me know how it goes !
maybe use some old computer speaker with integrated amplifier? 😉
Marks i tried your sketch and dont have sound in output i use the transistor 2N2222, and my speaker i use one speaker of the sound box computer, i have use one piezo speaker??
Isn’t it a problem that you’re logic level is at 5V whereas the SD card breakout wants only 3.3V logic?
Please help when starting the arduino uno it says that SD Fail in serial window. what is going wrong ?
Hi Mark,
I’m working on a project, and came across your page.
After I compile my sketch and write it to my arduino board, my serial monitor gives me a message saying “SD fail”…. I guess it’s not initializing the SD card, but do you have any idea what the problem is, and how to fix it?
Hi, thank you for this information, i was trying to do a similar thing except that with an SDHC card and not a micro SD….will the same connections do the job? i was trying to follow the equivalent connections of the ports by following the 4 bit SD bus mode found on http://en.wikipedia.org/wiki/Secure_Digital ….will that work?
thanks…..
It should work the same way as with MicroSD since they are basically the same thing.
okay,thanks for your reply…… i dont know why but when i connect D3 (SD pin 1) to the pin 10 of the arduino, the serial monitor shows sd fail…any suggestions?
Hi, just wanted to know, if i am using an SDHC card, what should i do with the unused pins? as of now with your specified connections, the card fails to read….please help!!!
My code refuses to compile giving the following errors:
C:UsersJamesDocumentsArduinolibrariesTMRpcmpcmRF.cpp:18: error: ‘RF24’ does not name a type
C:UsersJamesDocumentsArduinolibrariesTMRpcmpcmRF.cpp: In constructor ‘pcmRF::pcmRF(int, int)’:
C:UsersJamesDocumentsArduinolibrariesTMRpcmpcmRF.cpp:25: error: ‘RF24′ was not declared in this scope
C:UsersJamesDocumentsArduinolibrariesTMRpcmpcmRF.cpp:25: error: expected `;’ before ‘tmp’
C:UsersJamesDocumentsArduinolibrariesTMRpcmpcmRF.cpp:26: error: ‘radi’ was not declared in this scope
C:UsersJamesDocumentsArduinolibrariesTMRpcmpcmRF.cpp:26: error: ‘tmp’ was not declared in this scope
C:UsersJamesDocumentsArduinolibrariesTMRpcmpcmRF.cpp: In function ‘void checkRF()’:
C:UsersJamesDocumentsArduinolibrariesTMRpcmpcmRF.cpp:58: error: ‘radi’ was not declared in this scope
C:UsersJamesDocumentsArduinolibrariesTMRpcmpcmRF.cpp: In member function ‘void pcmRF::playRF(char*, int)’:
C:UsersJamesDocumentsArduinolibrariesTMRpcmpcmRF.cpp:126: error: ‘radi’ was not declared in this scope
Hello,
I want a device that plays 20 minutes of recorded music or voice from a SD card or USB chip. Then repeats the 20 minutes of music for months or years until stopped.
If you could do this, can you contact me?
Email or contact link at top of Blog.
Andy Graham
Hi! Unfortunately my focus is in a different domain right now… Sorry!
All you need is a 20-minute long wav file and the wav library shown above.
Code:
Follow the example sketches to initialize the library, then in the main loop:
if(!wav.isPlaying){
wav.play(“music.wav”);
}
pls write for me a code to interface an arduino uno and a card reader for logging data from a solar output voltage every half an hour
am new to programming
music:6: error: ‘TMRpcm’ does not name a type
??????
help me
do you know the pin assignments for the atmega2560? for the sd card? Im having a hard time plugging it up. thanks in advance.
hi how could i know the pin diagram of the sd card reader that you use because in my sd card there is a label GND, +3.3 , +5, CS, MOSI, SCK, MISO, GND. how could i know where is the D0 , D1 ,CLK, D3 in you pin assignments for the sd. thanks in advance
Here is the pin connections I found. I have not tried this project yet.
https://e47bdd65-a-bbf28792-s-sites.googlegroups.com/a/jo3ri.be/jo3ri/arduino/blogduino/castduinoaudioplayer/image.jpg?attachauth=ANoY7crHufEMbRBAFhpjPX6aW81KBzi-GhsqH3pVEU5Kw2smJKnhK0idC4LCTyrJodQJsjpkhnc33l4imt3jgcJ3a9TKbMCJRwU0aNLj9PTzg-Lbe8RUmkn1_9N_0YKL3Q_N8EmcLbDk-wd3dKGBjGes2mwqZgkkPYdd-Wj1U-Hd86RJ18GNjk8N83Bqm3qoYG9J6z1QenMc3xSJ8nnXvaP1RWqy61ShHD39O_-oliEipFdnm3Pvh5ch88p3rEi0CUi7Pp2MRjxp&attredirects=0
What modifications are necessary to play stereo files?
Unfortunately I don’t know what needs to be done in this case.
Read the readme file of the library, there’s a link to the wiki page.
hello@maks i am working on similar project like yours and using the same library. I have converted wav file as per your guidelines but still it wont work and if i try a code where just some tones are played it works fine. please help i am reaching deadline. i am using arduino uno and a sd card breakout board.
Hello! Unfortunately I won’t be able to help with this because I haven’t touched an arduino in a long time 🙁 I’m sorry
Had the same problem with “SD fail” … I found out that I was connecting the wrong pin, in this manual is “D1 goes to pin 11” but actually its not D1 (this pin is on the card reader too but is wrong to connect it). The correct pin is MOSI(D1) – in front as CMD.
Thanks for sharing that!
awesome post! worked for me! But I am getting a high pitch noise along with the sound I intend to play! Can you kindly suggest a method to get rid of it? Any suggestions are welcome! Thank you!
Hello!
I am trying to use your code but I get:
music:9: error: ‘TMRpcm’ does not name a type
music.ino: In function ‘void setup()’:
music:15: error: ‘tmrpcm’ was not declared in this scope
music.ino: In function ‘void loop()’:
music:37: error: ‘tmrpcm’ was not declared in this scope
music:49: error: ‘tmrpcm’ was not declared in this scope
Any ideas!
Thank you for your work!
OK, got that solved! 😀
How did you place the transistor?
Hi Andre!
I used few day ago the same code.
Do you copied into the folder libraries of Arduino, the folder TMRpcm of github?
Hi!
It was my bad, I had copied it into a wrong subfolder.
After long hustle with no success to get WTV020 to play, my arduino finally plays sounds!
Thank you all!
Hey Thanks for making this all available. I’m having a problem adding the TMRpcm library. I copied the zip file from github like I usually do with any other library but the Arduino environment isn’t recognizing it as a library. I tried adding another library just to see if it would work and it added it just fine so I’m guessing there’s something with the TMRpcm file itself that isn’t right. Do you have any ideas about why this would be happening?
So, I have my board wired up exactly as you describe above, but when I use the TMRpcm example, the card fails to initialize. I controlled for a lot of things, like the default SD_Chip_Select_Pin variable, and I’m at a dead end.
Hmmm, I wonder why it’s not recognizing.
Maybe following the installation section of the wiki would help installing it?
https://github.com/TMRh20/TMRpcm/wiki
Sorry, I don’t know why it wouldn’t work…
Have you tried a different card to see maybe that specific card isn’t supported?
Great, that is very usefull to make our electronics shematics souding; I’ve prefered the “Hack-hack” sounds from Mars-attacks for my InMoov project, so do you have a link for a such sketch runing wihtout PC, just by pushing a button for example => lazy to make it. Like a pseudo MP3 reader for Arduino?
Great! I tried it with my arduino uno and it worked all fine. But when I try it with an ATmega32 (not ATmega328) I get loads of errors, does anyone know how to fix this?
Errors:
DocumentsArduinolibrariesTMRpcmTMRpcm.cpp:16: error: ‘ICIE1’ was not declared in this scope
DocumentsArduinolibrariesTMRpcmTMRpcm.cpp:99: error: expected unqualified-id before ‘volatile’
DocumentsArduinolibrariesTMRpcmTMRpcm.cpp:99: error: expected `)’ before ‘volatile’
DocumentsArduinolibrariesTMRpcmTMRpcm.cpp:99: error: expected `)’ before ‘volatile’
DocumentsArduinolibrariesTMRpcmTMRpcm.cpp:99: error: expected initializer before ‘volatile’
DocumentsArduinolibrariesTMRpcmTMRpcm.cpp: In member function ‘void TMRpcm::stopPlayback()’:
DocumentsArduinolibrariesTMRpcmTMRpcm.cpp:365: error: invalid types ‘volatile unsigned char[byte]’ for array subscript
DocumentsArduinolibrariesTMRpcmTMRpcm.cpp: In member function ‘void TMRpcm::pause()’:
DocumentsArduinolibrariesTMRpcmTMRpcm.cpp:385: error: invalid types ‘volatile unsigned char[byte]’ for array subscript
DocumentsArduinolibrariesTMRpcmTMRpcm.cpp:385: error: ‘ICIE1’ was not declared in this scope
DocumentsArduinolibrariesTMRpcmTMRpcm.cpp:391: error: invalid types ‘volatile unsigned char[byte]’ for array subscript
DocumentsArduinolibrariesTMRpcmTMRpcm.cpp: In member function ‘void TMRpcm::play(char*, long unsigned int)’:
DocumentsArduinolibrariesTMRpcmTMRpcm.cpp:532: error: invalid types ‘volatile unsigned char[byte]’ for array subscript
DocumentsArduinolibrariesTMRpcmTMRpcm.cpp: In function ‘void __vector_6()’:
DocumentsArduinolibrariesTMRpcmTMRpcm.cpp:581: error: invalid types ‘volatile unsigned char[byte]’ for array subscript
DocumentsArduinolibrariesTMRpcmTMRpcm.cpp:587: error: invalid types ‘volatile unsigned char[byte]’ for array subscript
DocumentsArduinolibrariesTMRpcmTMRpcm.cpp:588: error: invalid types ‘volatile unsigned char[byte]’ for array subscript
DocumentsArduinolibrariesTMRpcmTMRpcm.cpp:600: error: invalid types ‘volatile unsigned char[byte]’ for array subscript
DocumentsArduinolibrariesTMRpcmTMRpcm.cpp: In member function ‘void TMRpcm::disable()’:
DocumentsArduinolibrariesTMRpcmTMRpcm.cpp:698: error: invalid types ‘volatile unsigned char[byte]’ for array subscript
Hello, I’m using an arduino uno. I followed the tutorial and got the connection to the sd card, but instead of music I only hear noise.
cheers,
deive alves
Hi! Unfortunately I don’t have time right now to create this sketch.
Hello, I’m havin’ trouble with the microsd module with my arduino atmega 2560, it say’s “SD fail” can somebody enlighten me on this? thank you very much. 🙂
where is the file SPI.h ? it’s the only thing i cant get
SPI.h comes with Arduino software, it should be there already…
I’m not able to find it, can you help?
Thanks for this information, but what is the purpose of the D0, D1, and CLK connected to 12, 13, and 11 on the arduino, respectively. I noticed they are not called out in the code anywhere so just curious as to what they do/why are they necessary (if they are)
Add #include to your sketch and it’ll work
I was wondering if there is a way to pass the filename as a variable instead of a character string? I have a whole series of short WAV files that I would like to play serially under normal conditions, but at times would like to play them randomly. If I could build an array of filenames and then simply pass the array entry in tmrpcm.play() that would be great. Something like tmrpcm.play(arrayNames[n]). Any ideas on how to do this short of modifying the library?
I downloaded everything per the instructions provided and everything has gone smooth except when I go to compile the sketch. I get an error that states TMRpcm.h: No such file or directory exists. Any ideas?
can help the problem?
It’s part of the SD library. The tmr library calls it within its own code that way its simplified when the user writes their code
Other sites say I need a logic level converter, is that necesary?
Is it possible to use TMRpcm with a sleep library? I’ve not had any luck so far.
Hi,
Maybe a silly question, but why is your wav file encoded at 16kHz and not 44100 Hz (the usual sampling frequency for wav file, I thought)? Is there a way to specify in the arduino code the sampling frequency of your sound?
Thanks 🙂
Gaelle
This is how you define an array of string literals:
char *filenames[] = {“foo”, “bar”};
I was getting a lot of noise so I turned off the library when a sound isn’t playing:
void loop(){
if (!tmrpcm.isPlaying()) {
tmrpcm.disable();
}
if(Serial.available()){
I didn’t find that necessary. I did connect the 3.3v pin on the sd card module to a real 3.3v power supply though. These modules are generally made for Arduino, so the board designers use various techniques to be able to tolerate/generate +5v-compatible signals even if the underlying hardware wants 3.3v.
That means you didn’t properly install the libraries that this sketch needs. See https://www.arduino.cc/en/Guide/Libraries under “Manual Installation” for how to do this in general. Specifically, the “libraries” folder in your Arduino installation should have a subfolder named “TMRpcm” and that folder should contain “TMRpcm.h” and several other files.
To find the root directory of the libraries folder, go to “File->Preferences” in your arduino application to see where the sketchbook location is. There should be a “libraries” subfolder under the sketchbook folder. If not, you can just create it and then copy the TMRpcm folder out of the zip file there.
This was a great sketch and explanation. I’ve used it to start a few projects that really worked perfect. But I have always had trouble playing ambient sounds. Music and words are great but even when I clean up the sound with hardware I still cant get waves crashing, whales taking or many synth sounds. I guess its an encoding problem but I’ve tried alot of combinations and just cant play those ambient humming sounds for outer space stuff
Did you ever get it working. That’s exactly my aim to reproduce that 3000 dollar led cloud that makes thunder and rain sounds. Would love to see some of your examples. I’m thinking of just investing in a proper mp3 shield.
TMRPCM’s upper sampling limit is 32kHz, IIRC. And back in the day, people often used 11kHz and 22kHz sampling frequencies. 😛
i cant transfer the filename using openNextFile() in sd library. it is to return the next filename in the path.. music.play(file.openNextFile())…this shows an error…also by transfering to a string and passing the filename
it can play wav file with high frequency ? and stereo wav file?
liked your dancing yoda 🙂 dont you need a DAC from the arduino to the speaker?
I have recorded my audio file as 512 bytes buffer ..can i play my 512 buffer audio with this code, but i have read somewhere that only 128 buffer audio can be played by using this TMRpcm library…
Will this work with and arduino pro mini?
I don’t see why it wouldn’t!
Thanks for this very help tutorial – now all my arduino projects can have sound for almost no cost!! WooHoo. Just a short note that might help others… my SD reader had the following pin configuration: CS (Chip select) -> pin10, SCK (Clock) -> pin13, MOSI (Data In) -> pin 11, MISO (Data Out) ->pin 12, VCC to 5V, GRD -> Ground. NOTE: The VCC MUST go to 5V – I originally connected it to the 3.3V and continually got the “SD Fail” message. Hope this helps others.
is it possible to read the SD card from an ethernet shield (or some other shield that can support SD cards)?
Curious, would it be possible to speed up or slow down the playback speed?
Hello, this worked fine but the volume is very low. I tried to add this line of code :
tmrpcm.setVolume (5);
I didnt make it 6 or 7 bec. when i do this the speaker just does noise but with 5 its good. So there is my question:
I want it to be louder than 5 with good audio quality. (Note: iam using 8 ohm 0.5W speaker)
How can I do this with arduino mega 2560 ?
Are you using a transistor or an amplifier for the speaker?
Hi, any idea how can I play a wave file at a certain pulse and freq?